prompt mine App
Find, Create & Share AI Magic
Implementing Machine Learning in Web Applications with Python Frameworks
Overview
This guide provides a comprehensive walkthrough of implementing machine learning algorithms in web applications using Python frameworks like Flask or Django. We'll cover the steps for model deployment, real-time predictions, and integrating APIs like TensorFlow.js for enhanced functionality.
Prerequisites
Familiarity with Python programming language
Basic understanding of machine learning concepts
Experience with Flask or Django frameworks
TensorFlow.js and scikit-learn libraries
Step 1: Model Development and Training
1. Choose a Problem: Identify a suitable problem for machine learning, such as image classification, text analysis, or predictive modeling.
2. Collect and Preprocess Data: Gather and preprocess relevant data, handling missing values and outliers as needed.
3. Split Data: Split the data into training (70-80%) and testing sets (20-30%).
4. Train a Model: Train a machine learning model using scikit-learn library, selecting an algorithm suitable for the problem.
5. Evaluate the Model: Evaluate the trained model using metrics such as accuracy, precision, recall, and F1-score.
Step 2: Model Deployment with Flask or Django
1. Choose a Framework: Select either Flask or Django for building the web application.
2. Create a New Project: Create a new project using the chosen framework.
3. Install Required Libraries: Install necessary libraries, including scikit-learn, TensorFlow.js, and Flask or Django.
4. Load the Trained Model: Load the trained machine learning model into the web application.
5. Create API Endpoints: Create API endpoints for handling incoming requests and returning predictions.
Example with Flask
python
from flask import Flask, request, jsonify
from sklearn.externals import joblib
app = Flask(__name__)
Load the trained model
model = joblib.load('model.pkl')
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
prediction = model.predict(data['features'])
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
Step 3: Real-Time Predictions
1. Create a Frontend: Create a frontend using HTML, CSS, and JavaScript to interact with the API endpoints.
2. Send Requests: Send requests to the API endpoints with input data.
3. Receive Predictions: Receive predictions from the API endpoints and display them in the frontend.
Example with JavaScript
javascript
fetch('/predict', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
features: [1, 2, 3, 4, 5]
})
})
.then(response => response.json())
.then(data => console.log(data.prediction));
Step 4: Integrating TensorFlow.js for Enhanced Functionality
1. Install TensorFlow.js: Install the TensorFlow.js library using npm or yarn.
2. Load the Model: Load the trained machine learning model into TensorFlow.js.
3. Use TensorFlow.js: Use TensorFlow.js to perform real-time predictions, visualizations, and other tasks.
Example with TensorFlow.js
javascript
const tf = require('@tensorflow/tfjs');
// Load the trained model
const model = await tf.loadLayersModel('model.json');
// Use the model for real-time predictions
const predictions = await model.predict(tf.tensor2d([1, 2, 3, 4, 5]));
console.log(predictions.arraySync());
Conclusion
Implementing machine learning algorithms in web applications using Python frameworks like Flask or Django can be a powerful way to build intelligent and interactive applications. By following the steps outlined in this guide, developers can deploy machine learning models, perform real-time predictions, and integrate APIs like TensorFlow.js for enhanced functionality.
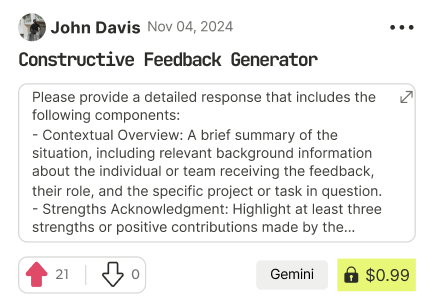
Find Powerful AI Prompts
Discover, create, and customize prompts with different models, from ChatGPT to Gemini in seconds
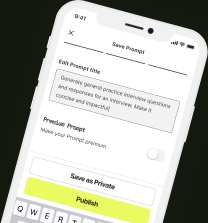
Simple Yet Powerful
Start with an idea and use expert prompts to bring your vision to life!