prompt mine App
Find, Create & Share AI Magic
Effective Error Handling in PHP: Strategies and Best Practices
Error handling is a crucial aspect of PHP development, as it enables developers to handle and recover from unexpected errors, exceptions, and unexpected behavior. In this response, we will discuss effective error handling strategies in PHP, including custom error handlers, logging frameworks, and graceful degradation. We will also cover best practices for debugging and error recovery in production environments.
Custom Error Handlers
Custom error handlers are user-defined functions that can be used to handle specific error types. These handlers can be used to perform actions such as logging, notification, or error reporting. Here's an example of a custom error handler:
php
function customErrorHandler($errno, $errstr, $errfile, $errline) {
// Log the error
error_log("Error [$errno] $errstr in $errfile on line $errline");
// Display a friendly error message to the user
echo "An unexpected error occurred. Please try again later.";
}
// Set the custom error handler
set_error_handler('customErrorHandler', E_ALL);
Logging Frameworks
Logging frameworks provide a more structured and organized way of handling errors and exceptions. Some popular logging frameworks for PHP include:
Monolog: A popular logging library that provides a flexible and extensible logging system.
Log4php: A logging library that provides a hierarchical logging system.
Here's an example of using Monolog to log errors:
php
use Monolog\Logger;
use Monolog\Handler\StreamHandler;
// Create a logger
$logger = new Logger('app');
// Create a stream handler to log to a file
$streamHandler = new StreamHandler('logs/error.log', Logger::ERROR);
// Add the stream handler to the logger
$logger->pushHandler($streamHandler);
// Log an error
$logger->error("An unexpected error occurred.");
Graceful Degradation
Graceful degradation is a technique that involves providing a fallback solution when an error occurs. This ensures that the application remains functional and provides a better user experience. Here's an example of using a fallback solution:
php
try {
// Perform an action that may fail
$result = doSomethingThatMayFail();
// Display the result
echo $result;
} catch (Exception $e) {
// Log the error
error_log("Error: " . $e->getMessage());
// Display a fallback solution
echo "Sorry, an unexpected error occurred. Please try again later.";
}
Best Practices for Debugging and Error Recovery
1. Use a logging framework: Logging frameworks provide a more structured and organized way of handling errors and exceptions.
2. Implement a custom error handler: Custom error handlers can be used to handle specific error types and perform actions such as logging and notification.
3. Use a fallback solution: Fallback solutions ensure that the application remains functional and provides a better user experience when an error occurs.
4. Test thoroughly: Thorough testing can help identify and fix errors before they occur in production.
5. Monitor application logs: Monitoring application logs can help identify errors and exceptions that may have occurred in production.
6. Implement a retry mechanism: Retry mechanisms can be used to retry failed actions and reduce the likelihood of errors occurring.
7. Keep sensitive information secure: When logging errors, make sure to keep sensitive information such as database credentials and API keys secure.
By implementing these strategies and best practices, developers can create robust and reliable applications that handle errors and exceptions effectively.
Example Use Cases
Handling errors in a web application: In a web application, errors can occur due to various reasons such as database connection failures, invalid user input, or unexpected exceptions. By implementing a custom error handler and logging framework, developers can handle errors and exceptions effectively and provide a better user experience.
Handling errors in a CLI application: In a CLI application, errors can occur due to various reasons such as invalid user input, unexpected exceptions, or system resource limitations. By implementing a custom error handler and logging framework, developers can handle errors and exceptions effectively and provide a better user experience.
Conclusion
In conclusion, effective error handling is crucial for creating robust and reliable applications. By implementing custom error handlers, logging frameworks, and fallback solutions, developers can handle errors and exceptions effectively and provide a better user experience. Additionally, by following best practices for debugging and error recovery, developers can identify and fix errors before they occur in production.
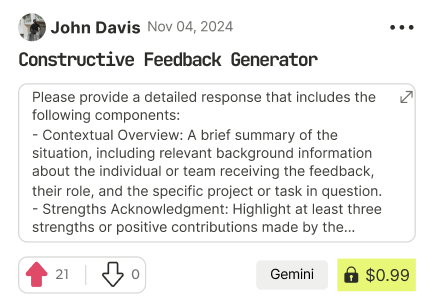
Find Powerful AI Prompts
Discover, create, and customize prompts with different models, from ChatGPT to Gemini in seconds
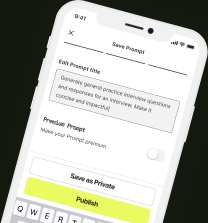
Simple Yet Powerful
Start with an idea and use expert prompts to bring your vision to life!