prompt mine App
Find, Create & Share AI Magic
Debugging JavaScript Functions: A Step-by-Step Guide
Debugging JavaScript functions can be a challenging task, especially when dealing with complex applications. To effectively identify and resolve issues, it's essential to follow a structured approach. This guide provides a step-by-step debugging process for JavaScript functions, covering the use of browser developer consoles, testing libraries, and techniques for resolving issues related to scope, async operations, and data handling.
Step 1: Identify the Issue
Clearly define the problem you're experiencing, including the expected result and the actual output.
Review the function's documentation and ensure you're using it correctly.
Verify that the issue is reproducible and not an isolated incident.
Step 2: Use Browser Developer Consoles
Open the browser developer console (e.g., Chrome DevTools, Firefox Developer Edition) and navigate to the Sources or Debugger tab.
Set breakpoints in your JavaScript code to pause execution and inspect variables.
Use the console.log() function to output variable values and debug messages.
Utilize the console's built-in functions, such as console.table() and console.group(), to format and organize your output.
Step 3: Isolate the Problem Area
Use the call stack to identify the sequence of function calls leading up to the issue.
Inspect the local scope and closure variables to ensure they're correctly defined and accessible.
Review the function's dependencies and ensure they're properly loaded and initialized.
Step 4: Test with a Testing Library
Choose a testing library (e.g., Jest, Mocha) and write unit tests to isolate the problematic function.
Use the library's assertion functions (e.g., expect()) to verify the function's behavior.
Run the tests and analyze the results to identify the root cause of the issue.
Step 5: Resolve Scope-Related Issues
Verify that variables are declared and accessed within the correct scope.
Use the let and const keywords to declare block-scoped variables.
Avoid using var declarations, which can lead to scope-related issues.
Use the this keyword correctly, ensuring it references the intended object.
Step 6: Handle Async Operations
Verify that async functions are correctly declared and called.
Use async/await syntax to simplify asynchronous code and improve readability.
Ensure that promises are properly handled and resolved.
Use try-catch blocks to catch and handle errors.
Step 7: Resolve Data Handling Issues
Verify that data is correctly formatted and passed to the function.
Use console.log() to inspect the data and ensure it matches expectations.
Validate user input and sanitize data to prevent security vulnerabilities.
Use data validation libraries (e.g., Joi) to enforce data consistency.
Step 8: Refactor and Optimize
Refactor the function to improve readability, maintainability, and performance.
Optimize the function for performance, using techniques such as memoization and caching.
Consider using a code linter (e.g., ESLint) to enforce coding standards and detect potential issues.
Example Use Case
Suppose you have a JavaScript function that's not returning the expected result:
javascript
function calculateTotal(prices) {
let total = 0;
prices.forEach((price) => {
total += price;
});
return total;
}
To debug this function, you would follow the steps outlined above:
1. Identify the issue: The function is not returning the expected total.
2. Use the browser developer console: Set a breakpoint and inspect the prices variable.
3. Isolate the problem area: Review the function's dependencies and ensure they're properly loaded.
4. Test with a testing library: Write unit tests to verify the function's behavior.
5. Resolve scope-related issues: Verify that the total variable is declared within the correct scope.
6. Handle async operations: None applicable in this example.
7. Resolve data handling issues: Verify that the prices array is correctly formatted and passed to the function.
8. Refactor and optimize: Refactor the function to improve readability and maintainability.
By following this step-by-step debugging process, you'll be able to identify and resolve issues related to scope, async operations, and data handling, ensuring your JavaScript functions return the expected results.
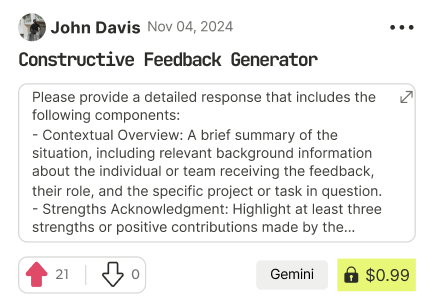
Find Powerful AI Prompts
Discover, create, and customize prompts with different models, from ChatGPT to Gemini in seconds
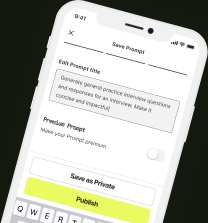
Simple Yet Powerful
Start with an idea and use expert prompts to bring your vision to life!