prompt mine App
Find, Create & Share AI Magic
Debugging JavaScript Code: A Guide
Okay, I'm ready to help you debug your JavaScript code. Please paste the code you want me to analyze.
Once you paste the code, I will:
1. **Analyze the code:** I'll read through your code carefully to identify potential errors, including syntax errors, logical errors, runtime errors, and potential performance issues.
2. **Explain the errors in detail:** I will provide a clear explanation of each error I find. This will include:
* The type of error (e.g., syntax error, type error, reference error, logical error).
* The line number where the error occurs (if applicable).
* Why the error is happening.
* The potential consequences of the error.
3. **Provide corrected code:** I will provide a corrected version of your code that fixes the identified errors.
4. **Explain the changes I made:** I will explain exactly what I changed in the code and why those changes were necessary to resolve the errors.
5. **Suggest best practices:** I will provide suggestions for best practices to help you avoid these types of errors in the future. This might include:
* Using a linter.
* Writing unit tests.
* Using TypeScript.
* Improving your understanding of JavaScript concepts.
* Using debugging tools.
* Practicing defensive programming.
I'm looking forward to helping you improve your code! Just paste it in.
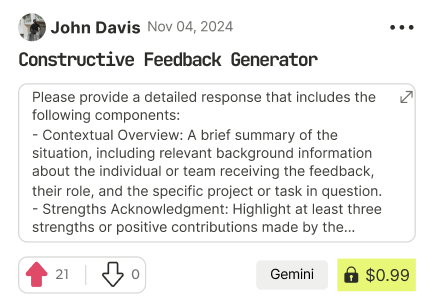
Find Powerful AI Prompts
Discover, create, and customize prompts with different models, from ChatGPT to Gemini in seconds
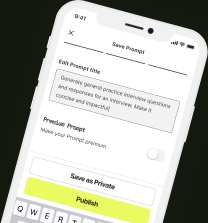
Simple Yet Powerful
Start with an idea and use expert prompts to bring your vision to life!